String Pool in Java
- Madhu Reddy
- Dec 15, 2019
- 2 min read
String class is one of the most important class in java.
It is widely used in every java-based application.
One of the Most Important questions on String during interviews is "what is the difference between String literal and new String ()? or what is String pool?"
Let’s look at them here.
String sLiteral="java Notes";
String sObject= new String("java Notes");
Both the statements above will create the String Objects.
but there is a difference in both the statements.
sLiteral will be stored in String pool. whereas sObject will be stored in heap memory.
Statement 1 will check if there is any String with the same value? if yes it will just give the reference of that String, otherwise it will create a new String in the String pool.
whereas statement 2 will create a new String in the heap memory no matter what.
hence the sLiteral and sObject will be pointing to different memory reference since one is created in String pool where as other one in heap memory.
String sLiteral="java Notes";
(since this is the first literal we are creating it will created in String pool)
String sLiteral2="java Notes";
(since already a String with the same value is there in the String pool reference of "sLiteral" will be returned instead of creating a new Object)
String sObject= new String("java Notes"); ( a new object is created in heap memory no matter what)
String sObject2= new String("java Notes");
( even though a String with the same value is created already, it will still create a new object in heap memory)
sLiteral==sLiteral2. //true since they are literals with same value both are referenced to the same memory in String pool.
sObject==sObject2. //false. since both are new String objects they will be referenced to two different memory locations.
sLiteral==sObject. //false. since one is literal and other is new object.
Note: "==" checks for the memory reference of the two objects.
where are String literals values stored ??
These are stored in a cache called as String pool.
In string pool each and every time an instance of the object is not created for the same string values. It just gives the reference of the existing value.
In versions prior to java 1.7, String pool is stored in Perm gen space which has a limited memory of 64 MB. Perm gen space was not available for garbage collection by JVM. So, this causes a lot of out of memory issues.
Hence from Java 1.7 String pool is now stored in heap memory. Hence are eligible for garbage collection by the JVM.
Below Image shows how the above created Strings would have been stored in the String pool.
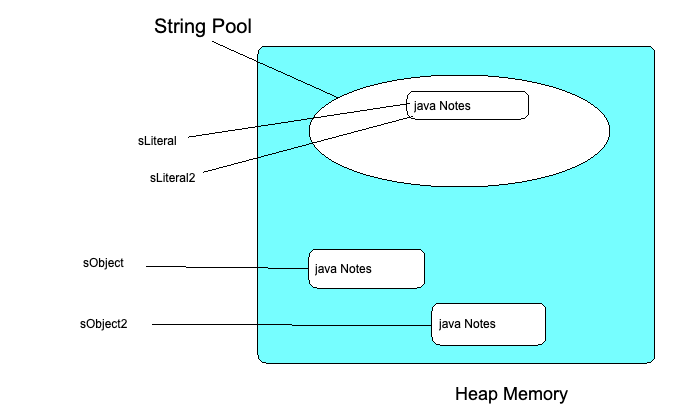
String Interning:
String objects created using new() operator can also be moved to String pool by interning.
by calling intern() on the String object it can be moved to String pool.
String sObject2= new String("java Notes");
sObject2.intern();
Now by calling intern() on sObject2 it will be now moved to String pool and the reference of literal will be given.
sLiteranl==sLiteral2==sObject2. //// true
Below image shows how the Strings now would be placed after calling intern() on sObject2.
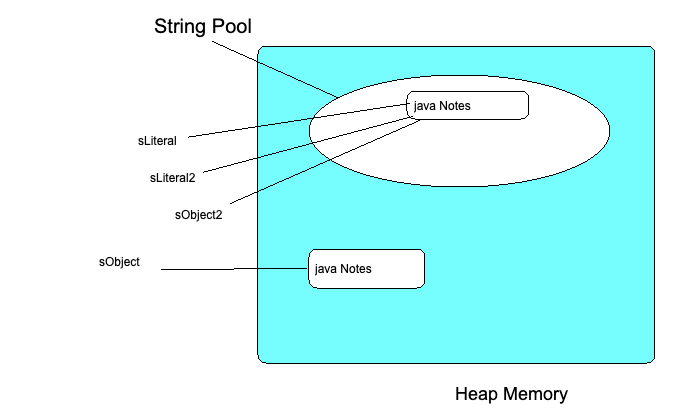
Comments